Pipes are the filters that are used to transform the data in angular applications.It can transform any type of data. It is denoted by the `|` symbol.
Create Command
ng generate pipe formatTime
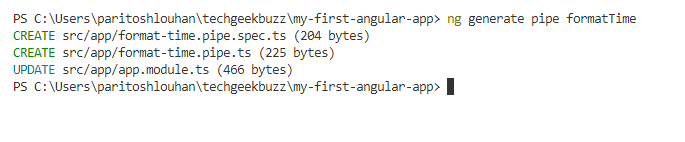
Here,
formatTime
is the pipe's name, which can be used to filter or format any set of data provided. Here formatTime is a custom pipe we created to demonstrate the command to create it. There are a lot of built-in pipes available in angular applications, which you can explore. Some of the names of built-in pipes are provided below.
1. Async pipe.
If data is an instance of observables, then the Async pipe subscribes to an observable and returns the transmitted values.
2. Currency pipe.
It converts a given number to any country's currency value format.
3. Slice pipe.
It slices a given array. It takes the index as an argument. We can assign a start and end index if you want to print a specific range of values.
4. Decimal pipe.
It is used to format decimal values.
5. Per cent pipe.
It formats the number as per cent.
6. Json pipe.
It is used to transform a js object into a JSON string.
Custom pipe
Custom pipe are the pipes we can create to filter our data. This is required most of the time as all available pipes cannot be used in all scenarios. In the example below, I have created formatTime pipe. It converts seconds to mm: ss format. Let's go through the source code.
format-time.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'formatTime'
})
export class FormatTimePipe implements PipeTransform {
transform(value: any, ...args: unknown[]): unknown {
return new Date(value * 1000).toISOString().substr(11, 8);;
}
}
html file
<h1>Total time remaining: {{totalSeconds | formatTime}}</h1>