A function is a container for a block of the code, that is used to perform a specific task. Using the function we can divide a big program into smaller modules or subparts. A function is like a small encapsulated block of code that can be called anywhere throughout the Program. There are two types of functions in JavaScript.
- User-defined function and,
- Built-in function
In the
User-defined function
, we define a function of our own and write the code that we want to perform with the function. And in this tutorial, we will learn only about JavaScript user-defined functions. There are also
built-in functions
in JavaScript such as
alert()
,
write()
,
prompt()
, etc. like a keyword(reserved variables) we have built-in functions.
Defining Functions in JavaScript
Defining a function in a JavaScript program is known as a
function definition
. Sometimes it is also referred to as
function declaration
or
function statement
. By defining a function means we write the block of code for the function that it needs to perform. To define a function we use the
function
keyword followed by the
- function name (a variable name to function)
- a list of parameters inside the parentheses separated by commas, and,
-
The JavaScript code inside the curly brackets
{......}
Syntax
function function_name(parameter1, paraneter2) { //function code block }
Example Now let's define a function that accepts a number, squares it and adds 10 to the number, and returns a value.
function sq_Add(number) { number = number*number; // square the number number +=10 ; //add 10 to number return number }On the first line, we are defining the function using the
function
keyword followed by the function name
sq_Add
and parameter
number
inside the parathesis
()
.
{}
we defined the function body, which is the procedure that this function should follow.
return
it. The
return
is a keyword, and it is used inside the function, to return a value. We will talk about the return statement in this tutorial subsequent section.
Calling a Function
In the above example, we saw how can we define a function in JavaScript. Defining a function is not enough, it's like writing instructions on paper, to execute a function we need to call it using its name followed by the parameters(if any) inside parentheses. We can call a function as many times as we want. This is the main reason why we write function, by defining a function once we can call it anywhere throughout.
Syntax
function_name(parameters...) //call function
Example Now let's call the function that we have defined in the above example.
<!DOCTYPE html> <html> <head> <title></title> <script> function sq_Add(number) { number = number*number; // square the number number +=10 ; //add 10 to number return number } let num1, num2; num1 = sq_Add(20); //function call num2 = sq_Add(10); //function call document.write("The value of num1 is-->"+num1); document.write("<br>") document.write("The value of num1 is-->"+num2); </script> <body> </body> </html>Output
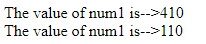
20
and
10
. In the above example, you can also see that we assign the function calling to the variables num1 and num2. This is because the function
sq_Add()
returns a value that can be stored in any variable.
JavaScript Function Parameter
A function may or may not have parameters. If we have defined parameters during function definition then we do need to pass the parameters while calling the function. The function parameters are the variables that are exclusive to the function, and we even cannot access a variable outside the function if it is defined inside the function. If a function has multiple parameters then, when we call the function we need to specify the same number of parameters, in the same order. Example
<script> function addnums(num1, num2, num3) { total = num1 + num2 + num3; return total } let result; let i= 20; let j=30; let k=50; result = addnums(i,j,k); document.write("The sum of i, j and k is--> "+result); </script>Output
The sum of i, j and k is--> 100Behind the code In the above example when we call the
addnums(i,j,k)
it calls the function definition
addnum(num1, num2, num3)
and set the value of num1 =i, num2=j and num3=k. In the function definition, we sum up all the three numbers num1, num2, and num3 and assign their sum to the
total
variable and
return
its value. Now let's see what the return statement does in a function.
Function return statement
The function may or may not have a return statement, it's optional. We generally use the return statement inside the function when we want the function to return a value. As we discussed above that we can not access the variable or values that are manipulated or created inside the function, that's why we use the return statement that returns a single value from the function. In the above example, we return the
total
variable, but in the background, the return statement returns back the value of the
total
that was the sum of three numbers. If a function does not have a return statement then by default it will return undefined. There is another fact about
return
statement, at moment the interpreter executes the return statement it though us out of the function with the return value.
Example
<script> function fun1() { return 1 } function fun2() { let n=1; //no return statement } function fun3() { return 3; //return only 3 return 4; // does not execute } let a, b , c; a= fun1(); b= fun2(); c= fun3(); document.write("The value of a is---> "+a); document.write("<br>"); document.write("The value of b is---> "+b); document.write("<br>"); document.write("The value of c is---> "+c); document.write("<br>"); </script>Output
The value of a is---> 1 The value of b is---> undefined The value of c is---> 3Behind the code In the above example the fun1() function return value 1, that's why when we assign the function to the variable
a
its value becomes 1. The
func2()
function has no return statement so it returns undefined, and with
b= fun2();
statement the
b
value became
undefined
. The func3() has 2 return statements, but when a function is executed the interpreter stops executing the function further when it encounters the
return
statement. The
return
statement is the
brake
for the function. In the above example when the JavaScript interpreter executes the
return 3
statement inside the function
func3()
it returns value 3 and gets back to the main code flow.
Summary
- A function is a container for the block of code, that is used to perform a specific task.
- The complete function use is divided into two steps function definition and function calling.
- To define a function we use the function keyword followed by the function name and parameters in parenthesis.
- To call a function we use its name and parameters in parenthesis.
- If we want a function to do some tasks and return some value back then we use the return statement inside the function.