What is an Operator in JavaScript?
An Operator can be a symbol, sign or keyword, that operates on operand (values) and return a result. For example * is an Arithmetic Multiplication operator, and it can be operated between two numerical operands(data values), 5*3 , and return the multiplication of 5 and 3 as a result. JavaScript supports major 7 types of Operators
- Arithmetic Operator
- Comparison Operator
- Logical Operator
- Assignment Operator
- Conditional Ternary operator
- Bitwise operator
- JavaScript Type Operator
1. Arithmetic Operators
As the operator name suggests, Arithmetic operators are borrowed from mathematics and they only work between two numbers data type values.
Arithmetic Operator | Description | Example | Result |
+ | Addition | 1+2; | 3 |
- | Subtraction | 1-2; | -1 |
* | Multiplication | 1*2; | 2 |
/ | Division | 1/2; | 0.5 |
** | Exponent | 2**3 | 8 |
% | Modulus (Remainder) | 13%4 | 1 |
++ | Increment | a=2; a++ | 3 |
-- | Decrement | a=2; a--; | 2 |
JavaScript Arithmetic Operators Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h1> JavaScript Arithmetic Operators</h1>"); let num1 = 20; let num2 = 3; document.write("<h3>num1 = 20, num2= 3 </h3>") document.write("<br>"); // line break document.write('num1 + num2 = '); document.write(num1+num2); document.write("<br>"); // line break document.write('num1 - num2 = '); document.write(num1-num2); document.write("<br>"); // line break document.write('num1 * num2 = '); document.write(num1*num2); document.write("<br>"); // line break document.write('num1 / num2 = '); document.write(num1/num2); document.write("<br>"); // line break document.write('num1 ** num2 = '); document.write(num1**num2); document.write("<br>"); // line break document.write('num1 % num2 = '); document.write(num1%num2); document.write("<br>"); // line break document.write('num1++ = '); num1++; // increment by 1 document.write(num1); document.write("<br>"); // line break document.write('num2-- = '); num2--; // decrement by 1 document.write(num2); document.write("<br>"); // line break </script> <body> </body> </html>Output:
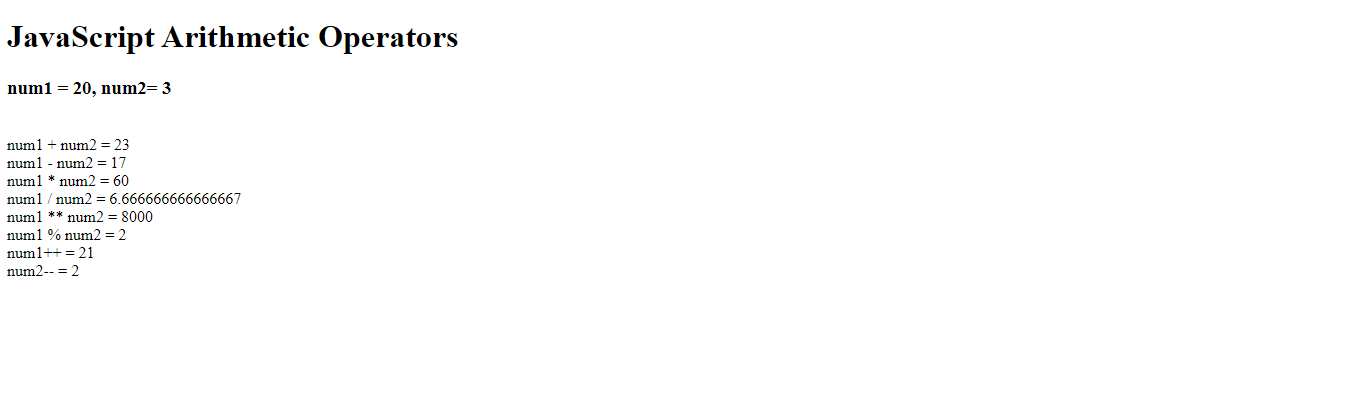
Note:
Arithmetic operator can only work between two numeric data values. If you use
+
operator between two strings or one string and one numeric value then insted of addition, concatination operation will be performed.
Example
document.write("one"+1);
the result will be
one1
2. Comparison Operators
The comparison operator also operates between two data values and return a boolean value
true
or
false
.
Comparison Operator | Description | Example | Result |
== | Equal | 1==2; | false |
!= | Not Equal | 1!=2; | true |
> | Greater than | 1>2; | false |
< | Less than | 1<2; | true |
>= | Greater than or equal to | 1>=2; | false |
<= | Less than or Equal to | 1<=2; | true |
Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h1> JavaScript Comparison Operators</h1>"); let num1 = 20; let num2 = 3; document.write("<h3>num1 = 20, num2= 3 </h3>") document.write("<br>"); // line break document.write('num1 == num2 = '); document.write(num1==num2); document.write("<br>"); // line break document.write('num1 != num2 = '); document.write(num1!= num2); document.write("<br>"); // line break document.write('num1 > num2 = '); document.write(num1>num2); document.write("<br>"); // line break document.write('num1 < num2 = '); document.write(num1<num2); document.write("<br>"); // line break document.write('num1 >= num2 = '); document.write(num1>=num2); document.write("<br>"); // line break document.write('num1 <= num2 = '); document.write(num1<=num2); document.write("<br>"); // line break </script> <body> </body> </html>Output
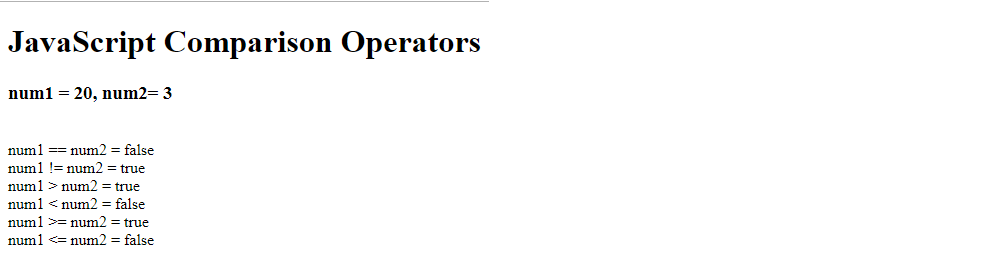
3. Logical Operators
The logical operator operates between two boolean values and also returns the result in a boolean value.
Logical Operator | Description | Example | Result |
&& | AND: The result will be true only if both the operands are true. | true && true | true |
|| | OR: the result will only be false if both the operands are false | true || false | true |
! | NOT: Reverse the boolean value. | ! true | false |
Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h1> JavaScript Logical Operators</h1>"); let a= true; let b=false; document.write("<h3>a = true, b= false </h3>") document.write("<br>"); // line break document.write('a && b = '); document.write(a && b); document.write("<br>"); // line break document.write('a || b = '); document.write(a||b); document.write("<br>"); // line break document.write('!a = '); document.write(!a); document.write("<br>"); // line break </script> <body> </body> </html>Output

4. Assignment Operators
The assignment operator is used to assign a value to a JavaScript variable. And when we use the Arithmetic operators with the assignment operators, it is known as Compound Assignment Operators in JavaScript.
Assignment Operator | Description | Example | Result |
= | Assignment operator | x =2; | x value will become 2 |
+= | Compound Addition Assignment Operator | x +=2; | x = x+2; |
-= | Compound Subtraction Assignment Operator | x -= 2; | x = x-2; |
*= | Compound Multiplication Assignment Operator | x *=2; | x = x*2; |
/= | Compound division Assignment Operator | x /=2; | x = x/2; |
% | Compound Modulus Assignment Operator | x %=2; | x = x%2; |
**= | Compound Exponent Assignment Operator | x **=2 | x = x**2 |
Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h1> JavaScript Assignment Operators</h1>"); let a=2; let b=3; document.write("<h3>a = 2, b= 3 </h3>") document.write("<br>"); // line break document.write('a += b = '); document.write(a += b); document.write("<br>"); // line break document.write('a -= b = '); document.write(a-=b); document.write("<br>"); // line break document.write('a *=b = '); document.write(a*=b); document.write("<br>"); // line break document.write('a /=b = '); document.write(a/=b); document.write("<br>"); // line break document.write('a **=b = '); document.write(a**=b); </script> <body> </body> </html>Output

5. Conditional Ternary Operator
The ternary operator is an expression which execution depends upon the true and false value. The ternary operator evaluates the expression and returns the appropriate result based on the true or false expression. It is called ternary operator because it takes three operands to work. The first operand is the condition The second operand is the true value. The third operand is the false value.
Ternary Operator | Description | Example | Result |
(condition) true value: false value | ternary operator | (a>b) x: y; | if a is greater than b return x else return y |
Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h1> JavaScript Ternary Operators</h1>"); let a=2; let b=3; document.write("<h3>a = 2, b= 3 </h3>") document.write("<br>"); // line break // ternary operator let c = (a>b)? 30 :40; document.write("c ="); document.write(c); </script> <body> </body> </html>Output

6. Bitwise Operators
The bitwise operator between two operands. The bitwise operator first convert the numeric data values to their corresponding 32-bit binary format then perform Bit operations between them.
Bitwise Operator | Description | Example | Result |
& | AND | 1 & 2 | 0 |
| | OR | 1|2 | 3 |
~ | NOT | ~ 1 | -2 |
^ | XOR | 1^2 | 3 |
<< | Zero Fill left shift | 1<<2 | 4 |
>> | Signed right Shift | 1>>2 | 0 |
>>> | Zero fill right shift | 1>>>2 | 0 |
Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h1> JavaScript Bitwise Operators</h1>"); let a=2; let b=3; document.write("<h3>a = 2, b= 3 </h3>") document.write("<br>"); // line break document.write("a & b ="); document.write(a & b ); document.write("<br>"); // line break document.write("a | b ="); document.write(a | b ); document.write("<br>"); // line break document.write("~a ="); document.write(~a); document.write("<br>"); // line break document.write("a ^ b ="); document.write(a ^ b ); document.write("<br>"); // line break document.write("a << b ="); document.write(a << b ); document.write("<br>"); // line break document.write("a >> b ="); document.write(a >> b ); document.write("<br>"); // line break document.write("a >>> b ="); document.write(a >>> b ); document.write("<br>"); // line break </script> <body> </body> </html>
Output
7. Type Operators
There are two Type operators in JavaScript
Type Operator | Description | Example | Result |
typeof | Return the Data Type of the operand. | typeof 20; | number |
instanceof | Return boolean value true or false if the variable is an instance of the object. | a = {name:"value"}; a instanceof Object; | true |
Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h1> JavaScript Type Operators</h1>"); let a = {name:"Rahul", age:20}; document.write("typeof a = "); document.write(typeof a); document.write("<br>") document.write("a instanceof Object= "); document.write(a instanceof Object); </script> <body> </body> </html>Output
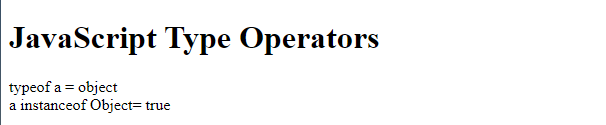
Summary
- Operators are used to perform operations between operands
- The operator could be a binary, unary, or ternary operator.
- JavaScript support 7 different types of Operators.
- The Arithmetic Operators perform arithmetic operations between operands.
- The Comparision operator compares two operands and returns a boolean value.
- The Logical operator performs operation between the boolean operands and also returns a boolean value.
- The assignment operator assigns a value to the variable.
- The ternary operator work between three operands.
- The bitwise operator converts the numeric value to 32 bits binary format and performs bit operation on the binary format.
- The typeof operator returns the Data Type of variable.
- The instanceof operator checks if the operand is an instance of the specified object.
People are also reading: