The JavaScript code cannot execute standalone, it needs to be embedded in the HTML file inside the HTML
<script>
tag.
JavaScript Syntax
There are two ways, to embed a JavaScript code in an HTML file.
- Internal JavaScript
- External JavaScript
Internal JavaScript Code
In the Internal JavaScript code, we write the complete JavaScript code inside the HTML document between the opening
<script>
and closing
</script>
tags. And an HTML file can have more than 1 <script> tags. We Generally use Internal JavaScript when we want to put all the code in the same HTML file. And Internal JavaScript is only preferred when you are working on a small front-end project.
Example
#js-syntax.html
<!DOCTYPE html> <html> <head> <title></title> <script> alert("JavaScript Syntax")</script> </head> <body> <h1>Syntax Tutorial Internel JavaScript</h1> </body> </html>As the browser parse the HTML document from top to bottom, that's why the
<script>
tag will execute before the
<body>
tag. When you execute the above HTML file in your web-browser you will see a similar output.
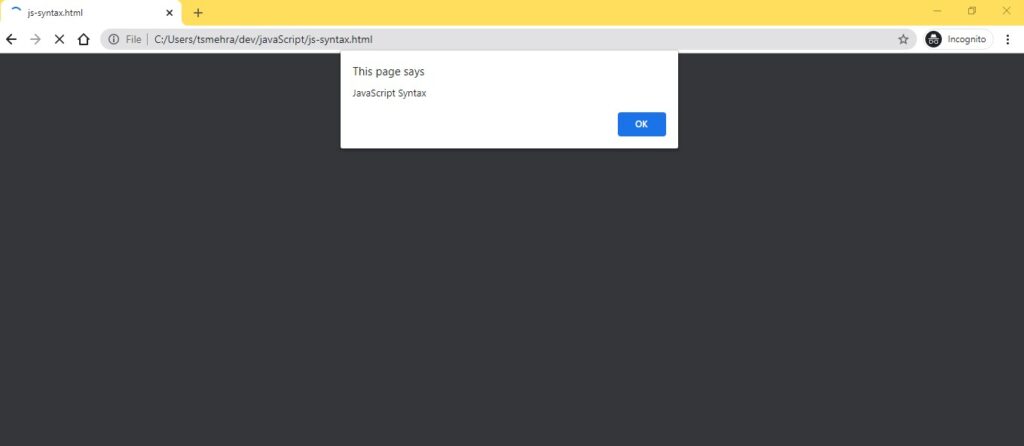
External JavaScript
In external JavaScript, we create a separate JavaScript file with the file extension
.js
. In the separate JavaScript file, we write all the JavaScript code and import that file in our HTML file using
<script>
tag and
src
attribute.
Example
#js-external.js
alert("JavaScript Syntax External")#js-syntax.html
<!DOCTYPE html> <html> <head> <title></title> <!-- External JavaScript --> <script src="js-external.js"> </script> </head> <body> <h1>JavaScript Syntax Tutorial External JavaScript</h1> </body> </html>The Internal JavaScript and External JavaScript will work the same, but when you work on big projects, there you need to keep your project modular. In that case, you need to create separate JavaScript files for JavaScript code.
Placement of the Script Tag:
<script> in the <head> tag
<!DOCTYPE html> <html> <head> <title></title> <!-- Script Tag in the Head --> <script src="js-external.js"> </script> </head> <body> <h1>JavaScript Syntax Tutorial</h1> </body> </html>
<script> in the <body> tag
In most professional websites, you will see that all the <script> tags are located in the <body> element, and at the bottom. This is because there JavaScipt might need to manipulate an HTML element, which can only happen if the complete <body> element is loaded before the JavaScript code, else you might see some error.<!DOCTYPE html> <html> <head> <title></title> </head> <body> <h1>JavaScript Syntax Tutorial</h1> <!-- Script Tag in the Body --> <script src="js-external.js"> </script> </body> </html>
Script Element Attributes
The <script> Element supports 8 attributes
- async (only for External Script)
- crossorigin
- defer (only for External Script)
- integrity
- nomodule
- refferpolicy
- src
- type
async
The async attribute can only be used with External JavaScript <script> tag. It is a boolean value, which runs the script asynchronously as soon as the browser parses the Script.
<script src="js-external.js" async> </script>
crossorigin
The
crossorigin
attribute is used when we are importing the JavaScript file from another server.
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/js/bootstrap.bundle.min.js" integrity="sha384-ygbV9kiqUc6oa4msXn9868pTtWMgiQaeYH7/t7LECLbyPA2x65Kgf80OJFdroafW" crossorigin="anonymous"> </script>
defer
defer
attribute is the opposite of
async
attribute, it is also a boolean attribute but it does not execute or run the page until the complete HTML page gets parsed by the browser.
defer
attribute only works with external Script.
<script src="js-external.js" defer> </script>
integrity
The
integrity
attribute contains the
filehash
value which allows the browser to check the fetched script.
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/js/bootstrap.bundle.min.js" integrity="sha384-ygbV9kiqUc6oa4msXn9868pTtWMgiQaeYH7/t7LECLbyPA2x65Kgf80OJFdroafW" crossorigin="anonymous"></script>
nomodule
The nomodule is a boolean attribute that represents whether or not to execute the Script in the browser that supports ES2015 modules.<script nomodule> alert("Hello")</script>
referrerpolicy
The
reffererpolicy
attribute represent which referrer information to send when the script is fetched.
<script src="js-external.js" referrerpolicy="origin"> </script>
src
The
src
attribute represents the source location or URL for the external JavaScript.
<script src="js-external.js" > </script>
type
The type attribute defines the media type of the script.
<script type="text/javascript"></script>
Case Sensitivity
JavaScript is a case-sensitive programming language. It supports the concepts of keywords, identifiers, functions, and objects which make more sense if the programming language is case sensitive. By case-sensitive programming language, we mean we can not use different case staying to access the same object, for example
alert()
will prompt up an alert box but
ALERT()
will throw an error.
Comments in JavaScript
Comments are used to provide extra information about the code itself, the browser interpreter does not execute the comment. There are two ways we can write comments in JavaScript code.
- Single Line Comment
- Multi-Line Comment
To write a single line comment we use the double backslash symbol // Example
<script> //this is a single line comment </script>
/*
symbol and ends with
*/
symbol
<script> /* This is a multi line comment */ </script>
Summary
- JavaScript needs to be embedded or imported into the HTML file.
- We can use JavaScript in an HTML file with two methods, Internal and External.
-
In External JavaScript we write the separate JavaScript code in
.js
file. - JavaScript is a CaseSenstive Programming Language.
-
//
is used to write single-line comments and /* & */ are used to write multi-line comments in JavaScript.
People are also reading: