In the previous two JavaScript tutorials, we discuss how can we use the
if…else
and
switch case
statements in JavaScript to execute a specific block of code based on single and multiple conditions. In this tutorial, we will learn about the concept of
while loop in JavaScript
which can execute a block of code again and again based on the true condition.
Many times while writing a program we encounter some situations where we want to execute a block of code repeatedly until the specific condition becomes false. And this can be done with the help of while loops in JavaScript. There are two types of while loops in JavaScript
- while loop
- do-while loop
JavaScript while Loop
Although there are many types of statements in JavaScript that can perform looping structure, among them
while
is the most basic and commonly used loop. We generally used
while
loop
when we are not certain about the number of loop iterations, and where the looping depends on a condition.
Flow Chart
In the above while loop flow chart you can see that, the block of while loop code will keep on executing till the condition of while loop is true. In case if the while loop condition remains true, you will encounter an infinite loop, where the while loop code block keeps executing until you close the browser. An infinite loop is a bug that you do not want to be in your program so be careful with the condition you put with while loop. If the condition is false, to begin with, the code block of the while loop will not execute at all.
JavaScript while loop Syntax
In JavaScript, we follow the following syntax to implement a while loop.
while (conditional expression) { //while loop code }
the conditional expression could be a boolean value or an expression returning a boolean value.
JavaScript while loop Example
Here is the loop real use case example of JavaScript while loop
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h3> JavaScript While Loop Example</h3>"); let countdown =10; while(countdown>0) { document.write(countdown); document.write("<br>"); //break line //decrease countdown value countdown=countdown-1; } </script> <body> </body> </html>
Output
From the above output, you can see that till the while loop condition
countdown
value is greater than
0
the while loop code block keeps executing. And when its value became
0
the condition
countdown>0
became
false
and the while loop code block did not execute further.
JavaScript do-while Loop
The
do...while
loop in JavaScript is similar to the
while
loop. The only difference is, in
while
loop the condition is checked before the code block execution but in
do...while
the condition is checked after the block execution. This is because of the placement of the condition, in
while
loop the condition is above the while code block, but in
do..while
the condition is below the do..while code block. Becaue of this below condition placement, the code block of
do..while
executes at least one time even the condition is false to start with.
do...while Flow Chart
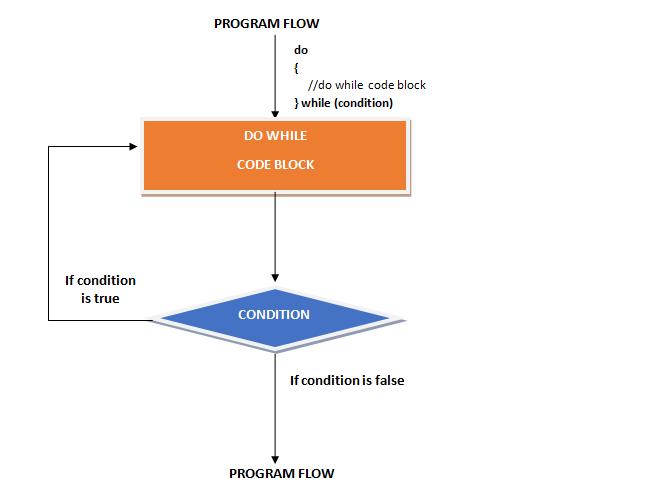
do....while Syntax
To implement
do..while
in JavaScript, we follow the following syntax
do { //do while code block }while(condition)From the above syntax and flow chart, you can see that the code block is before the while condition. This means the the JavaScript interpreter will execute the code first then go for the condition check, because of the top to bottom approach.
JavaScript do...while loop Example
<!DOCTYPE html> <html> <head> <title></title> <script> document.write("<h3> JavaScript Do While Loop Example</h3>"); let countdown =10; do { document.write(countdown); document.write("<br>"); //break line //decrease countdown value countdown=countdown-1; }while(countdown>0) </script> <body> </body> </html>Output
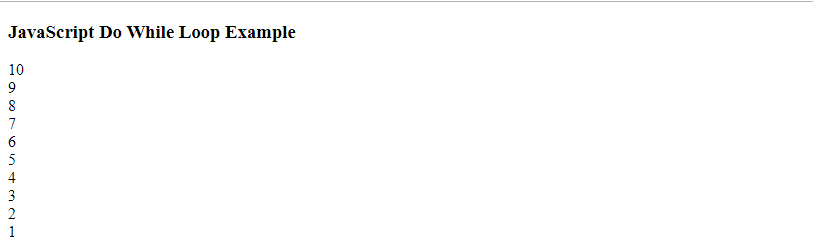
while
loop will not print any output, but
do..while
loop will print
0.
Summary
- while is a loop statement that executes the same block of code again and again till the condition becomes false.
- If the condition never becomes false the while loop will keep executing the code till eternity.
- The do..while loop is similar to the while loop, but in do..while the condition is checked after the code block execution.
-
If the condition is
false
from the start thewhile
loop code block will not execute but thedo..while
code block will execute at least once.
People are also reading: